API
ResourceSpace implements a RESTful API that returns JSON. All requests must be signed using a shared private key specific to each user. This can be performed via GET or POST.
To make an API call simply access the /api/ URL of your ResourceSpace installation with the following parameters:
user | The alphanumeric ID of the user to access the system as, for example "admin". Use the function urlencode (or similar) on any usernames that contain special characters such as @ or use the encode value within the string. | |
---|---|---|
function | The function you wish to perform. See the API function reference for more details. | |
param1, param2, param3... | The parameters to pass to each function. These are specific to each function and are documented in the function reference. It's important that the values are properly percent-encoded. Parameters can be passed by name. This allows requests to miss optional parameters if default is OK. Use the function urlencode (or similar) on any parameter that contain special characters such as @ | ) or use the encode value within the string. | |
sign | The signature. In order to ensure API requests are not 'tampered with' during transmission we require the caller to build a signature string. This is generated by taking the API key (Session or user) then appending the query URL, and finally producing a sha256 hexadecimal hash of that string. | |
authmode | The authentication mode. This must be the last parameter passed and can be set to one of the following:-
From v10.2, native authmode requires a valid CSRF token to be POSTed for state changing operations. If failing to provide one, ResourceSpace will return an appropriate error message. The CSRF token should be provided using the configurable identifier. |
Examples
- GET example
- POST example
- Get a session key using a password
- Updating resource metadata
- Using the API test tool
Basic GET example
This is a GET example in PHP.
// Set the private API key for the user (from the user account page) and the user we're accessing the system as.
$private_key="bfab0581232784c7b04a5c9";
$user="admin";
// Search for 'cat'
$query="user=" . $user . "&function=do_search&search=cat";
// Sign the query using the private key
$sign=hash("sha256",$private_key . $query);
// Make the request and output the JSON results.
echo file_get_contents("https://my.resourcespace.system/api/?" . $query . "&sign=" . $sign);
The equivalent in Perl:
#!/usr/bin/perl -w
use Digest::SHA qw(sha256_hex);
use LWP::Simple;
use Data::Dumper;
use JSON;
use strict;
# Set the private API key for the user (from the user account page) and the user we're accessing the system as.
my $private_key = "bfab0581232784c7b04a5c9";
my $user = "admin";
# Search for 'cat'
my $query = "user=$user&function=do_search&search=cat";
# Sign the query using the private key
my $sign = sha256_hex($private_key . $query);
# Make the request and output the JSON results.
my $result = decode_json(get("https://my.resourcespace.system/api/index.php?$query&sign=$sign"));
print Dumper($result)
private_key="bfab0581232784c7b04a5c9";
query='user=admin&function=do_search&search=cat';
sign=$(echo -n "${private_key}${query}" | openssl dgst -sha256);
curl -X POST "https://my.resourcespace.system/api/?${query}&sign=$(echo ${sign} | sed 's/^.* //')"
This is a POST example in PHP.
// Set the URL to point to API.
$url = "https://my.resourcespace.system/api/";
// Formulate the API query data.
$data = array(
'user' => $user,
'function' => 'do_search',
'param1' => 'test',
'param2' => '1,2',
'param3' => 'date',
'param4' => '0',
'param5' => '4',
'param6' => ''
);
$query = http_build_query($data);
// Sign the query using the private key.
$sign=hash("sha256",$private_key . $query);
// This part simply replicates the GET query string to be POSTed as a single 'query' POST item.
$data['sign'] = $sign;
$postdata = array();
$postdata['query'] = http_build_query($data);;
$postdata['sign'] = $sign;
$postdata['user'] = $user;
$curl = curl_init($url);
curl_setopt( $curl, CURLOPT_HEADER, "Content-Type:application/x-www-form-urlencoded" );
curl_setopt( $curl, CURLOPT_POST, 1);
curl_setopt( $curl, CURLOPT_POSTFIELDS, $postdata);
curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1 );
// Make the request and output the results.
$curl_response = curl_exec($curl);
print_r(json_decode($curl_response));
Getting a session key
This is an example of using GET to obtain a session API key in PHP.
// Set the username and password
$username="auser1";
$password="mypassword";
// Set the query to call the login() function, passing in the username and password.
$query="function=login&username=" . $username . "&password=" . $password;
// Make the request and output the session API Key. There is no need to sign as we don't have a key yet.
echo file_get_contents("https://my.resourcespace.system/api/?" . $query);
Updating resource metadata
For fixed list fields, you can use a combination of get_field_options() and update_field() as below.
Your code will probably need to do the following:-
- Retrieve all available options for the field using get_field_options()*
- Check the array to find the node IDs of the values you want to set
- Use update_field() to set the required node options for the required resource
* If the field is a dynamic tag field you can use set_node() with $returnexisting set to true to obtain the required node ID. This will return an existing node value if it already exists for that field, or will create a new node ID if not
// Set the username and password $user="abby.cee"; $private_key="f45c204eb831fec99a867681c22d7bfab0581232784c7b04a5c9"; // Get the valid options for field 75 (emotion) by calling get_field_options(). Setting $nodeinfo=true returns complete node data $data = array( 'user' => $user, 'function' => 'get_field_options', 'ref' => '75', 'nodeinfo' => true, ); $query = http_build_query($data); $sign=hash("sha256",$private_key . $query); $data['sign'] = $sign; $postdata = array(); $postdata['query'] = http_build_query($data);; $postdata['sign'] = $sign; $postdata['user'] = $user; $curl = curl_init($url); curl_setopt( $curl, CURLOPT_HEADER, "Content-Type:application/x-www-form-urlencoded" ); curl_setopt( $curl, CURLOPT_POST, 1); curl_setopt( $curl, CURLOPT_POSTFIELDS, $postdata); curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1 ); $curl_response = curl_exec($curl); $fieldoptions = json_decode($curl_response,true)); // This will populate the $fieldoptions variable as below ( [0] => Array ( [ref] => 267 [name] => Happy [parent] => 0 => 10 [translated_name] => Happy ) [1] => Array ( [ref] => 268 [name] => Sad [parent] => 0 => 20 [translated_name] => Sad ) [2] => Array ( [ref] => 269 [name] => Inspirational [parent] => 0 => 30 [translated_name] => Inspirational ) [3] => Array ( [ref] => 270 [name] => Eye contact [parent] => 0 => 40 [translated_name] => Eye contact ) [4] => Array ( [ref] => 271 [name] => Thoughtful [parent] => 0 => 50 [translated_name] => Thoughtful ) // Now use update_field()to set the nodes with ID's 269 ('Inspirational') and 270 ('Eye contact') for resource #4206 $data = array( 'user' => $user, 'function' => 'update_field', 'resource' => '4206', 'field' => '75', 'value' => '269,270', 'nodevalues' => true, ); $query = http_build_query($data); $sign=hash("sha256",$private_key . $query); $data['sign'] = $sign; $postdata = array(); $postdata['query'] = http_build_query($data); $postdata['sign'] = $sign; $postdata['user'] = $user; $curl = curl_init($url); curl_setopt( $curl, CURLOPT_HEADER, "Content-Type:application/x-www-form-urlencoded" ); curl_setopt( $curl, CURLOPT_POST, 1); curl_setopt( $curl, CURLOPT_POSTFIELDS, $postdata); curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1 ); $curl_response = curl_exec($curl); $curl_error = curl_error($curl); print_r($curl_error); print_r($curl_response); echo "COMPLETE";
For fixed list fields you can simply use update_field() to set the required values as in the example below.
// Use update_field() to set the value for resource 1002, field 8 (Title) to 'New logo'
// Set the username and password
$user="abby.cee";
$private_key="f45c204eb831fec99a867681c22d7bfab0581232784c7b04a5c9";
$data = array(
'user' => $user,
'function' => 'update_field',
'resource' => '1002',
'field' => '8',
'value' => 'New logo',
);
$query = http_build_query($data);
$sign=hash("sha256",$private_key . $query);
$data['sign'] = $sign;
$postdata = array();
$postdata['query'] = http_build_query($data);
$postdata['sign'] = $sign;
$postdata['user'] = $user;
$curl = curl_init($url);
curl_setopt( $curl, CURLOPT_HEADER, "Content-Type:application/x-www-form-urlencoded" );
curl_setopt( $curl, CURLOPT_POST, 1);
curl_setopt( $curl, CURLOPT_POSTFIELDS, $postdata);
curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1 );
$curl_response = curl_exec($curl);
$curl_error = curl_error($curl);
print_r($curl_error);
print_r($curl_response);
echo "COMPLETE";
Other languages are possible and would work in a similar way.
API test tool
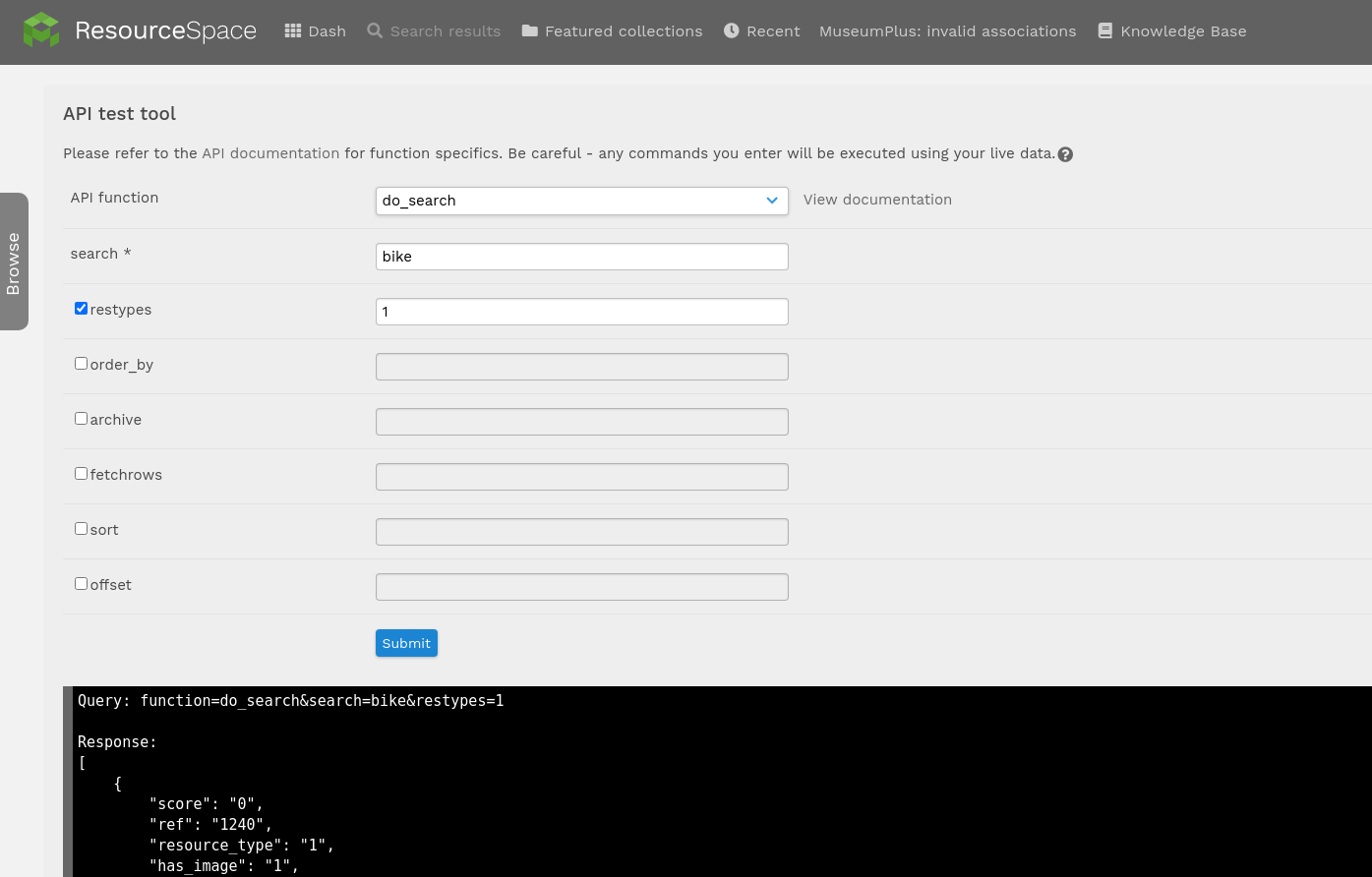
The API test tool (Admin -> System -> API test tool) allows you to try out commands directly in the ResourceSpace interface prior to inclusion in your development environment.
Simply select the function then enter the parameters as defined in the API documentation. The JSON output is displayed below. Furthermore, the tool will output working PHP and cURL code for you as a starting point for your integration.
Any optional arguments must be actively enabled in order to be applied when submitting the request.
Enabling and Disabling the API
The ResourceSpace API is enabled by default, however it can be disabled by changing the enable_remote_apis config option in config.php
$enable_remote_apis = false;
Disabling the API will hide the API Test tool, API secret keys on the user records and prevents all API calls from working unless the "native" authmode is used as this is required for the ResourceSpace user interface.